2 releases
0.1.1 | Oct 20, 2024 |
---|---|
0.1.0 | May 22, 2021 |
#27 in #kraken
447 downloads per month
39KB
485 lines
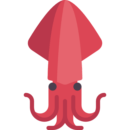
Calamari is a REST API client for Kraken.
Quickstart
The API client comes in two flavors: PublicApiClient
and PrivateApiClient
.
The former has access to the public methods only, the latter to all endpoints.
This is enforced at compile-time, as all the endpoints are defined statically
in the traits PublicEndpoints
and PrivateEndpoints
.
use calamari::{PublicApiClient, PublicEndpoints};
// Note: to run this example you will need to add Tokio to your dependencies:
// tokio = { version = "1.0", features = ["macros", "rt-multi-thread"] }
#[tokio::main]
async fn main() {
let client = PublicApiClient::default();
println!("Server time: {}", client.time().await.unwrap());
println!("System status: {}", client.system_status().await.unwrap());
println!("Ticker: {}", client.ticker("pair=XBTUSD".into()).await.unwrap());
}
Each endpoint accepts either zero arguments or a single argument containing all
the request parameters in urlencode format. All endpoints return a String
containing the JSON response from the server, leaving the user with complete
freedom in how they want to handle it.
A PrivateApiClient
can be instantiated directly, or created from an existing
PublicApiClient
by supplying the API credentials with the set_credentials
method.
use calamari::{ApiCredentials, PrivateApiClient, PublicEndpoints, PrivateEndpoints};
#[tokio::main]
async fn main() {
let credentials = ApiCredentials::new(
"YOUR_API_KEY".into(),
"YOUR_API_SECRET".into(),
);
let client = PrivateApiClient::default_with_credentials(credentials);
// Alternatively, if `client` is already a `PublicApiClient`:
// let client = client.set_credentials(credentials);
println!("Server time: {}", client.time().await.unwrap());
println!("System status: {}", client.system_status().await.unwrap());
println!("Ticker: {}", client.ticker("pair=XBTUSD".into()).await.unwrap());
println!("Account balance: {}", client.balance().await.unwrap());
println!("Open orders: {}", client.open_orders("trades=true".into()).await.unwrap());
}
Documentation
The complete documentation is available on docs.rs.
Dependencies
~5–16MB
~196K SLoC